Ever since I started writing code, I always wondered- How these code linters, static code analysers, and code that helps you create code (e.g. angular cli module generator, create-react-app) works? This curiosity of mine helped me to explore a wonderful data structure called ‘AST’ or ‘Abstract Syntax Tree’.
So what is an AST?
According to its Wikipedia Definition- ‘’In computer science, an Abstract Syntax Tree (AST), or just Syntax Tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code.’’
Or, in simpler words, it is a data structure or to be very specific- A tree data structure where each node of the tree will give us information about the Code such as variable declaration, statement, branching, etc.
But before going in detail of AST, allow me to quickly tell you what you will get after reading this blog post series. Yes, you heard it right ‘Series’. It is a four-part series where we will be exploring -
- What is AST? Some basic details,
- Writing simple NodeJs scripts that will help you to analyse & modify your code on the fly,
- Writing custom Eslint rules, and
- Writing code to write code (but not in a scary sci-fi-ai way).
As you will be able to guess by now that the whole series will be around Javascript Ecosystem. But the knowledge acquired here can be used for other platforms too (or Not !! I am not really sure 🤔 But It will be fun). And now it is time to start some useful discussion. So buckle up 🚀 …
As Wikipedia already told you, AST stands for Abstract Syntax Tree. So let me quickly break down this full form. AST for a code simply means representation of the syntactic structure of that code in the form of a tree but in a very abstract way. By abstract, it means it may not contain all the information of your source code. Some inessential information can be skipped while building an AST, this information could be semicolon, braces, parentheses, etc. But all other essential information is present in the tree such as variable types, the order of execution, LR components of binary operations, etc.
Let’s see how does a simple AST look like-
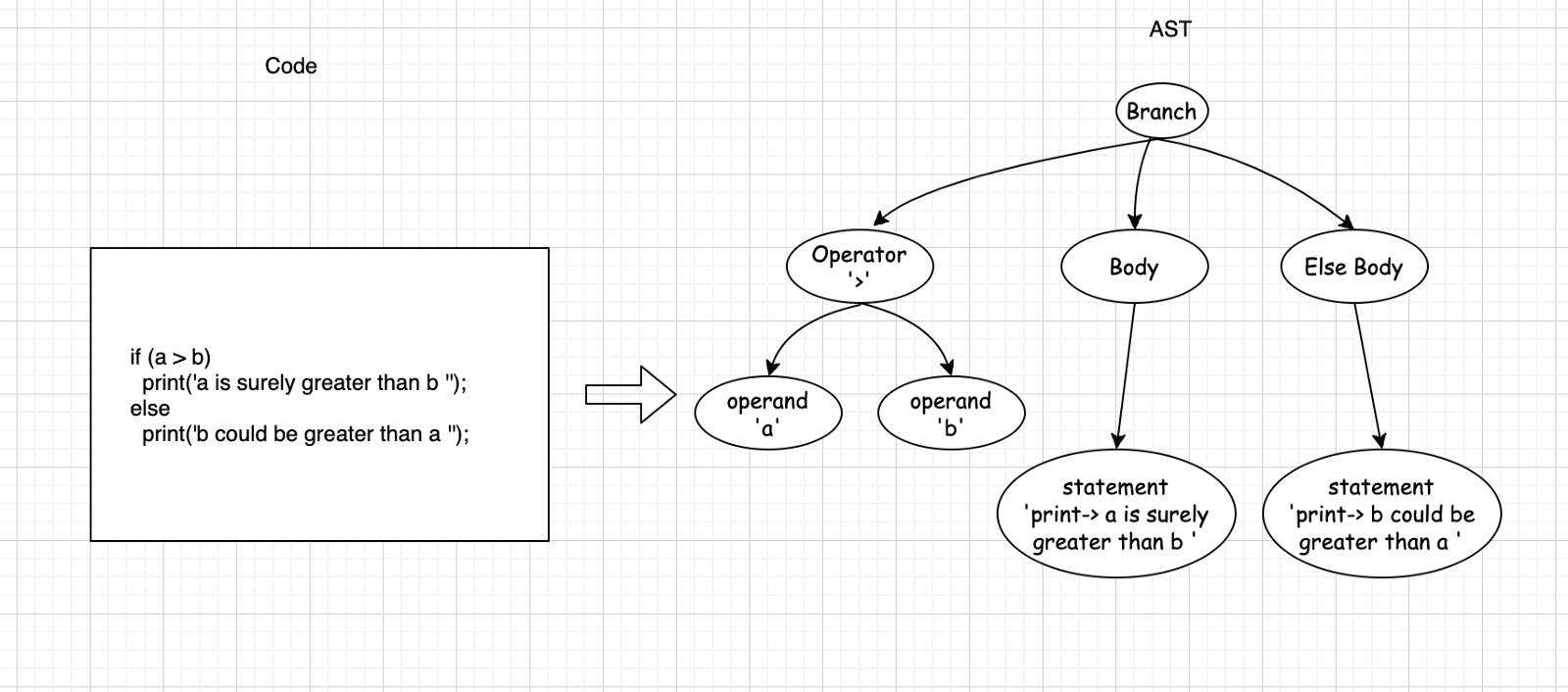
Here we can see that we have a ‘if statement’ and its corresponding syntax tree and for single if statement we have a node called ‘branch’ and it’s children representing various construct of an ‘if statement’ like condition, body and else body (Note that this AST is only for example purpose, AST generated from real parser contains much more information).
Using AST in Javascript Ecosystem
To use AST in javascript you will need few tools. Here is a list of some tools that you can use for parsing, traversing and reconstruction -
1. Esprima
You remember the parsing thingy I was talking about, means converting your code to an AST. Yeah, this Esprima fellow helps you do that. And it’s super simple. You just need to npm install esprima
, import it into your script, pass your code as an argument and tada. See so simple -

Hmm, now you will be wondering, the AST seems quite complex to traverse and performing operation onto it would be little( exhaustively high 😫 😫) difficult. But you know I won’t be writing this line if there was no solution for it. So allow me to present to you-
2. Estraverse
You can pass your generated AST to its traverse function and It will help you to go through every node. Estraverse provides you methods like enter and leave. Confused 😕 see below -
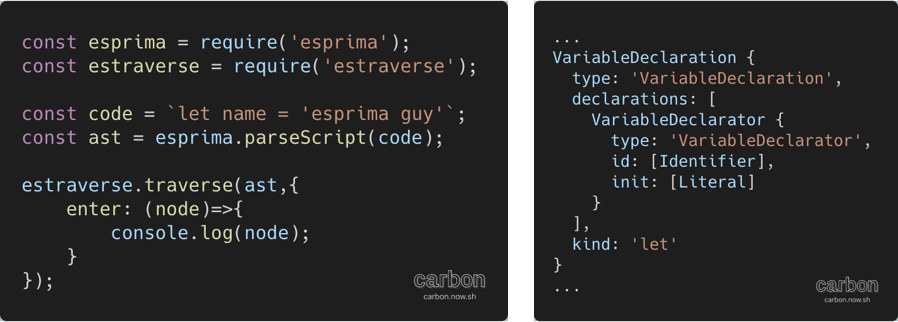
3. Escodegen
This is another very useful module in NodeJs ecosystem. Escodegen helps you generate code back from its AST. Let’s say you have modified something in the tree itself, then you can use this tool to generate new code from that modified tree. More use of this tool we will see in 2nd and last part of this series. For now, look at the example below-
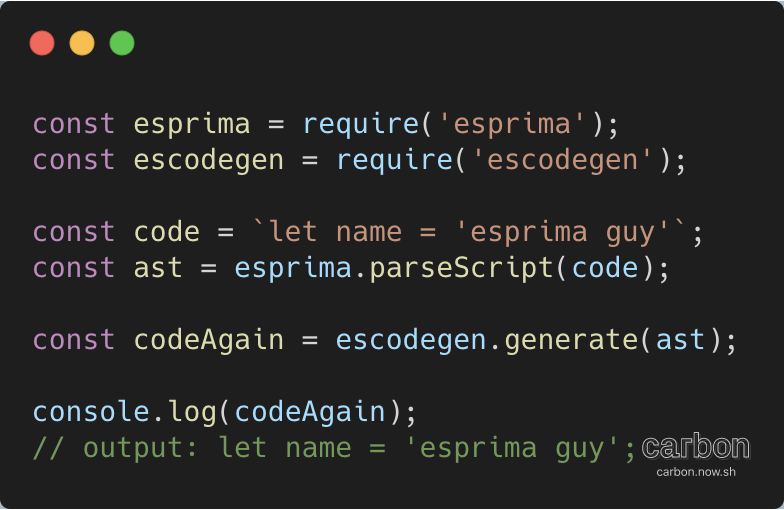
All the above tools are really helpful to work with AST in the Javascript environment. There are many other tools like these and provides a different set of functionality so check them out too.
In the next post, we will get into real action and will write scripts to analyse code and do modifications to the code. And yeah spoiler alert- We gonna slay some pythons 😈. That all for now. Other blogs in the series -
- (Next) Writing a Python Slayer in Javascript using AST
- (Next) Creating your very own custom ESLint plugin
- (Next) Dynamically generating code using AST
…, Over and Out.
Attributions:
- Photo by Lukasz Szmigiel on Unsplash
- Beautiful code snips by Carbon